Java Multidirectional Arrays
Hello there, fellow coder! Today, we’re diving into the world of Java Multidirectional Arrays. Buckle up, because this is going to be a fun ride!
Introduction
Ever wondered how to store data in a tabular form, like a table with rows and columns, in Java? Well, that’s where multidimensional arrays come into play. They’re like a single container that stores multiple containers. Cool, right?
Table of Contents
Understanding Java Multidimensional Arrays
A multidimensional array in Java is simply an array of arrays. Imagine it like a matrix, where each cell can store a value. It’s a powerful tool that can help you represent complex data structures, like a chessboard or a spreadsheet.
Declaring and Initializing Multidimensional Arrays in Java
Declaring a multidimensional array in Java is a piece of cake. Here’s the syntax:
dataType[][] arrayName;
JavaThis is how you tell Java that you want a multidimensional array. The dataType
can be any valid data type in Java, and arrayName
is the name you want to give to your array.
And initializing? Just as easy:
int[][] myArray = new int[3][3];
JavaThis creates a 3×3 array. You can also initialize it with values right away:
int[][] myArray = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
JavaThis creates a 3×3 array with the specified values.
Following diagram summarizes the two types of multidimensional arrays in Java.
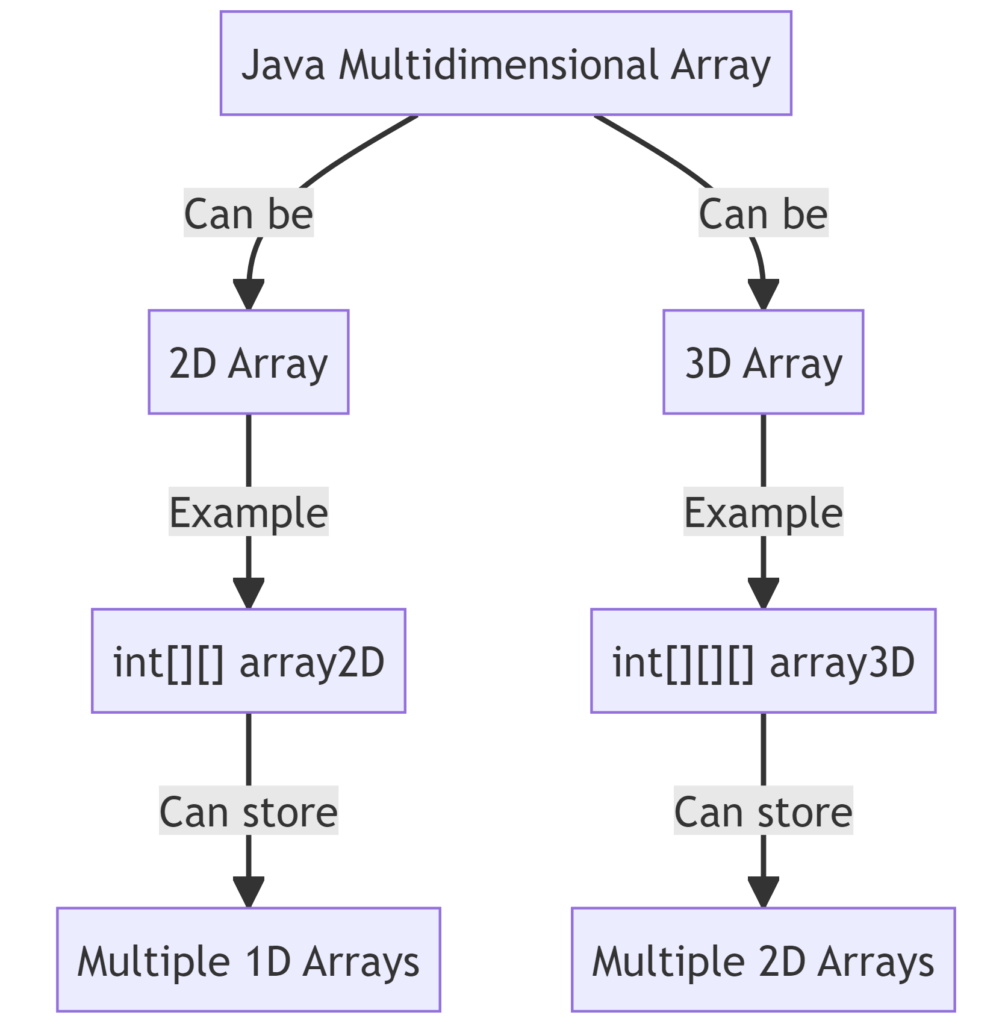
Accessing Elements in Multidimensional Arrays
Accessing elements in a multidimensional array is like going on a treasure hunt. You just need the right map (or in this case, indices). Here’s how:
int x = myArray[0][2]; // This will give you the value 3
JavaThis line of code retrieves the value at the first row and third column of the array, which is 3.
Manipulating Multidimensional Arrays in Java
Manipulating data in a multidimensional array is like playing with Lego blocks. You can change, add, or remove data as you please. Here’s an example:
myArray[1][1] = 10; // This changes the value at row 2 and column 2 to 10
JavaThis line of code changes the value at the second row and second column of the array to 10.
Multidimensional Arrays and Loops
Loops and multidimensional arrays go together like peanut butter and jelly. You can use loops to traverse through the array and perform operations. Here’s a simple example using a for loop:
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
System.out.print(myArray[i][j] + " ");
}
System.out.println();
}
JavaThis loop goes through each element in the array and prints it. The System.out.println();
is used to print a new line after each row.
Java Multidimensional Arrays Examples
Let’s put everything we’ve learned into practice with some code examples.
Code Example 1: Creating and Manipulating a Multidimensional Array
public class Main {
public static void main(String[] args) {
// Creating a 3x3 array
int[][] myArray = new int[3][3];
// Initializing the array with values
int value = 1;
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
myArray[i][j] = value;
value++;
}
}
// Printing the array
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
System.out.print(myArray[i][j] + " ");
}
System.out.println();
}
}
}
JavaThis code creates a 3×3 array and initializes it with values from 1 to 9. It then prints the array. The output will be:
1 2 3
4 5 6
7 8 9
Code Example 2: Using Loops with Multidimensional Arrays
public class Main {
public static void main(String[] args) {
// Creating and initializing a 3x3 array
int[][] myArray = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
// Using a loop to multiply each element by 2
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
myArray[i][j] *= 2;
}
}
// Printing the array
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
System.out.print(myArray[i][j] + " ");
}
System.out.println();
}
}
}
JavaThis code multiplies each element in the array by 2 and then prints the array. The output will be:
2 4 6
8 10 12
14 16 18
Conclusion
And that’s a wrap! You’ve just learned how to declare, initialize, access, and manipulate multidimensional arrays in Java. You’ve also learned how to use loops with multidimensional arrays. With this knowledge, you’re well on your way to becoming a Java pro!
Frequently Asked Questions
Why would you need a multidimensional array in Java?
Multidimensional arrays are useful when you want to store data in a tabular form, like a table with rows and columns.
How to check if an array is multidimensional in Java?
You can check the type of the array. If it’s an array of arrays, then it’s multidimensional.
Does Java have multidimensional arrays?
Yes, Java supports multidimensional arrays.
How to check if two multidimensional arrays are equal in Java?
You can use the Arrays.deepEquals()
method to check if two multidimensional arrays are equal.
Interview Questions (FAQ)
-
What is a multidimensional array in Java?
A multidimensional array in Java is an array of arrays. Each element of a multidimensional array is an array itself.
-
How do you declare and initialize a multidimensional array in Java?
A multidimensional array in Java is declared similarly to a single-dimensional array, but with additional square brackets for each additional dimension. For initialization, you can use nested curly braces, each containing elements of the sub-array.
-
How do you access elements in a multidimensional array?
Elements in a multidimensional array can be accessed by using their index numbers. For a two-dimensional array, we need two indices — one for the row and one for the column.
-
Can you explain the different steps of declaring multidimensional arrays in Java?
Declaring a multidimensional array involves specifying the type of the elements, followed by multiple sets of square brackets, each representing a dimension. Initialization can be done at the time of declaration or separately.
-
How do you use loops with multidimensional arrays in Java?
Loops can be used to traverse through the elements of a multidimensional array. A nested loop is typically used, with the outer loop iterating through the rows and the inner loop iterating through the columns.
-
What is ArrayStoreException in Java?
ArrayStoreException is an exception that is thrown to indicate that an attempt has been made to store the wrong type of object into an array of objects.
-
How to compare two multidimensional arrays in Java?
Two multidimensional arrays can be compared in Java using the
Arrays.deepEquals()
method. It returns true if the two specified arrays are deeply equal to one another. -
What are the different ways to create arrays in Java?
Arrays in Java can be created in several ways, including using the
new
keyword followed by the data type and array size, and using array literals with initialization lists. -
Can you pass the array to the method in Java?
Yes, you can pass an array to a method just like any other variable in Java. The method can then access and modify the elements of the array.
-
Can you store a String in an array of Integer in Java?
No, you cannot store a String in an array of Integer in Java because they are different data types. Attempting to do so would result in a compile-time error.
Related Tutorials
- Java Arrays: Single and Multi-Dimensional Array
- 2D Arrays in Java – Two-Dimensional and Nested Arrays
Happy coding!