Get Started with Java in the Easiest Way
Introduction to Java
Hello there! Ready to dive into the world of Java? Java is a powerful, object-oriented programming language used by millions of developers worldwide. It’s known for its simplicity, readability, and scalability. And the best part? Write your code once, and run it anywhere – that’s the beauty of Java.
Table of Contents
Setting Up Your Java Development Environment
Before we start coding, let’s set up our Java development environment. You can choose from various Integrated Development Environments (IDEs) like IntelliJ IDEA, Eclipse, or NetBeans. For this tutorial, we’ll use IntelliJ IDEA.
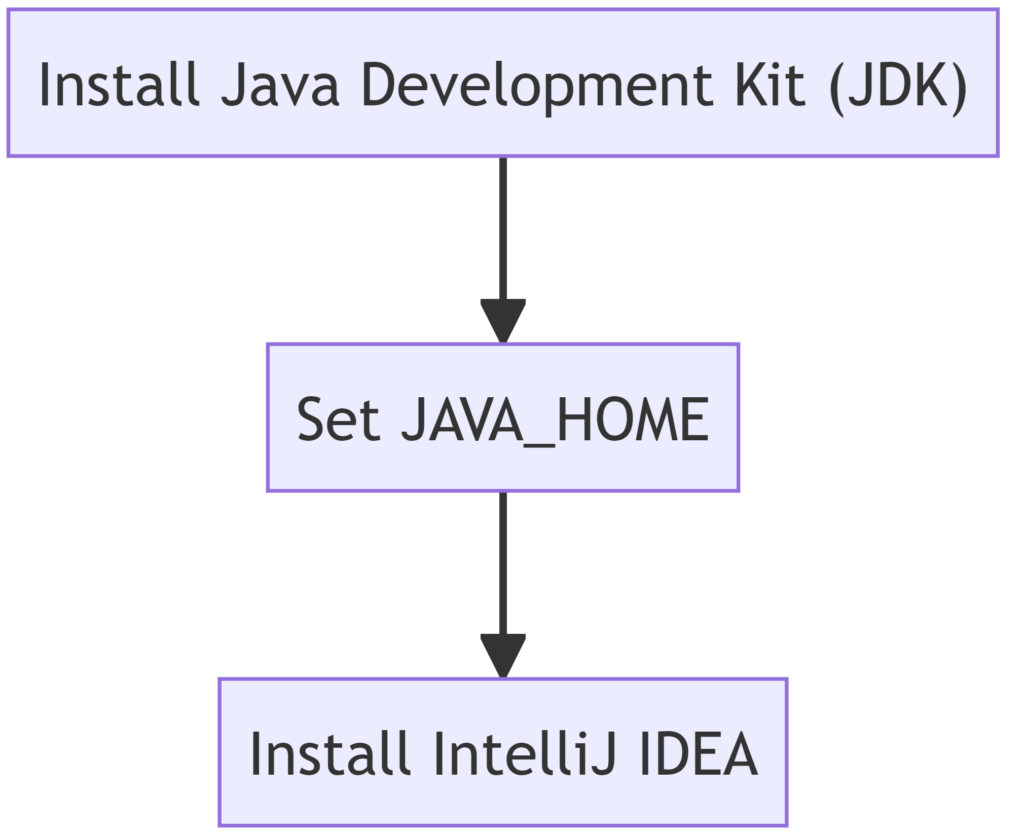
1. Download and install the Java Development Kit (JDK).


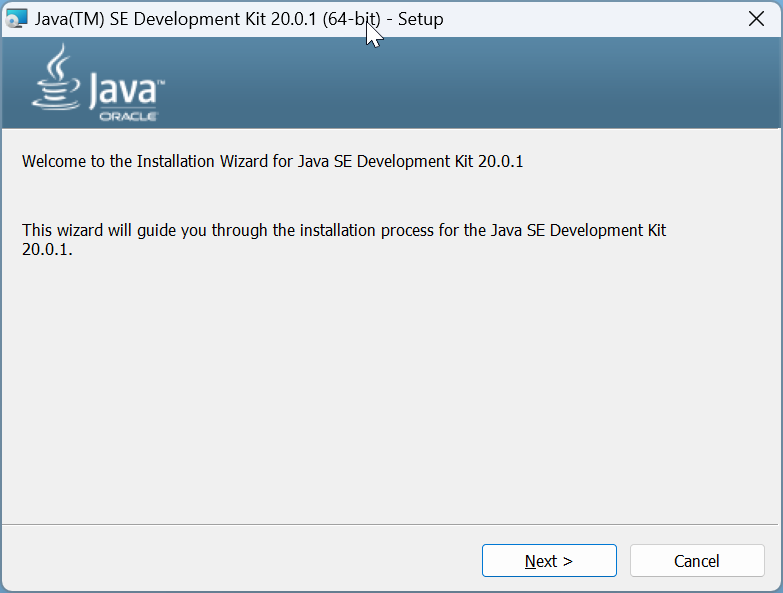
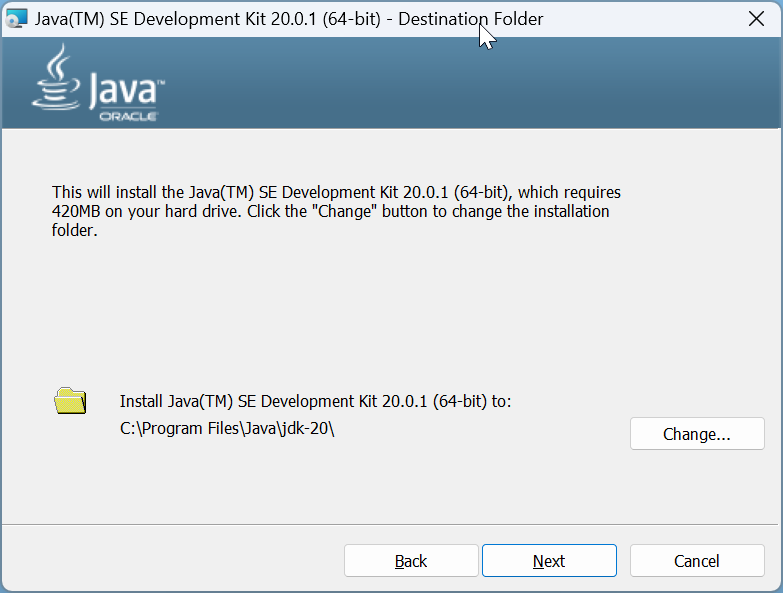

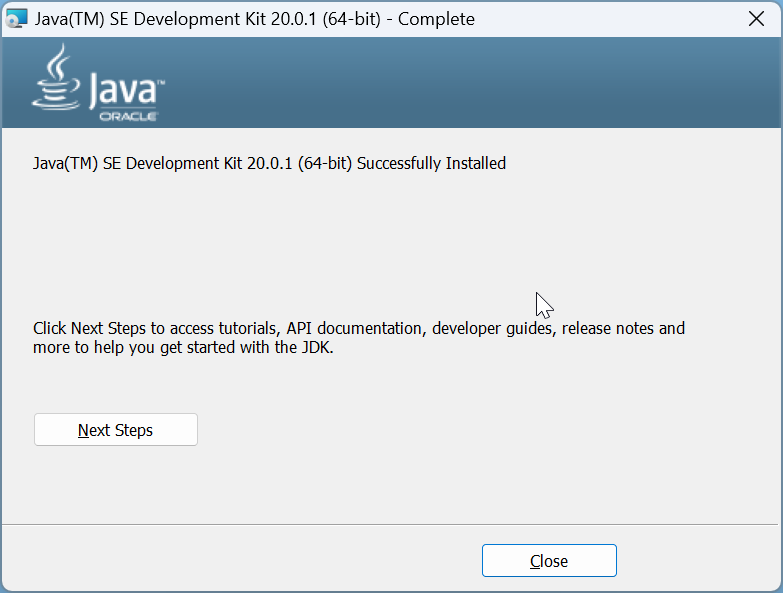
2. Set the JAVA_HOME
environment variable to point to the JDK installation directory.
Sure, I’d be happy to elaborate on that.
The JAVA_HOME
environment variable is used by some Java tools and applications to find the Java Development Kit (JDK) installation directory. It’s essentially a way for these tools to know where Java is installed on your computer.
Here’s how you can set the JAVA_HOME
environment variable:
On Windows:
- Find the path to the JDK installation directory. This is usually something like
C:\Program Files\Java\jdk-xx
, wherexx
is your installed JDK version.
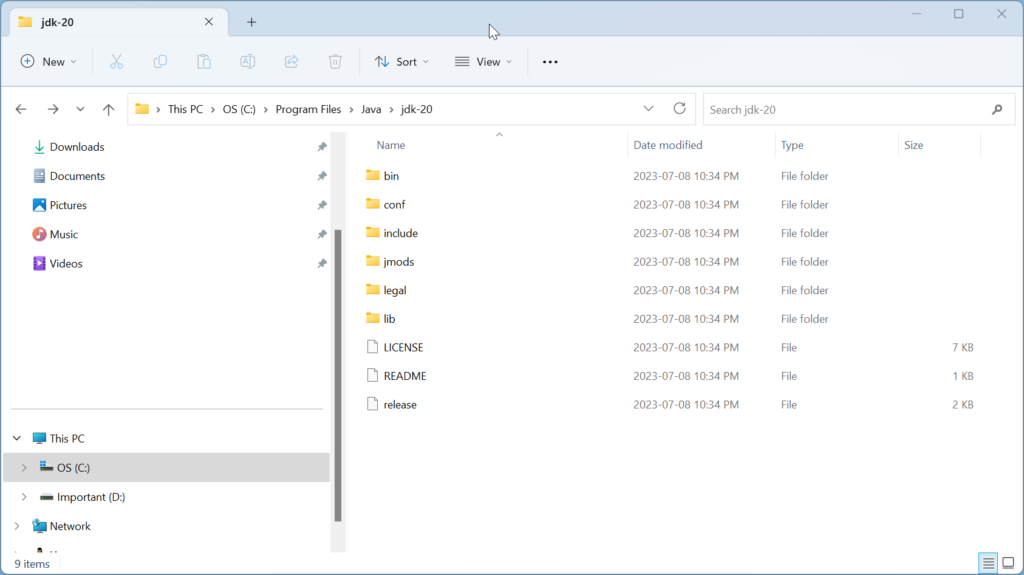
- In windows 10 or 11, search for Environment Variables then select Edit the system environment variables. In windows 7, Right click My Computer and select Properties > Advanced > Click the Environment Variables button.

- Click on ‘New’.

- Enter
JAVA_HOME
as the Variable name and the path to the JDK installation directory as the Variable value. - Click ‘OK’ to close all dialog boxes.
On macOS:
- Open Terminal.
- Enter the following command:
echo 'export JAVA_HOME=$(/usr/libexec/java_home)' >> ~/.bash_profile
- Restart Terminal or enter
source ~/.bash_profile
to reload your profile.
On Linux (bash shell):
- Open Terminal.
- Enter the following command:
echo 'export JAVA_HOME=/usr/lib/jvm/java-xx-openjdk-amd64' >> ~/.bashrc
, wherexx
is your installed JDK version. - Restart Terminal or enter
source ~/.bashrc
to reload your profile.
Remember to replace the paths in the above instructions with the actual path to your JDK installation directory.
3. Download and install IntelliJ IDEA (Free Community Edition)
IntelliJ IDEA is one of the most popular IDE for Java Programming and most Java Programmer use this and often recommends this.
On Windows:
- Download the free Community Edition of IntelliJ IDEA.

2. Once downloaded, double-click the IntelliJ IDEA installer file idealC-20xx.x.x.exe.

3. Click “Next” on IntelliJ IDEA Community Edition Setup Window. Click Next on the next windows as well.


4. In the next window, make sure to check the boxes to associate IntelliJ IDEA with .java
, .groovy
, and .kt
file. Press Next.
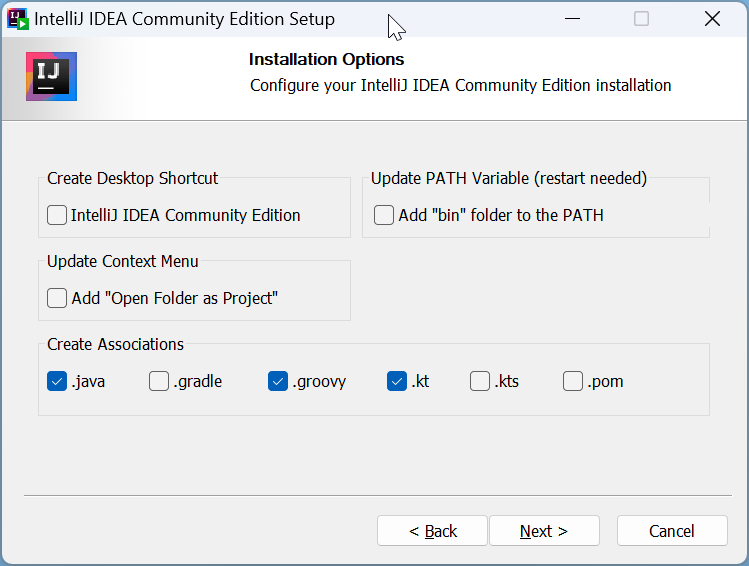
5. Follow instruction. Click Install.



- On macOS:
- Open the downloaded
.dmg
file. - Drag and drop the IntelliJ IDEA app file into the Applications folder.
- Open the downloaded
- On Linux:
- Unpack the downloaded
.tar.gz
file using the command:tar -xzf idea-xx.tar.gz
, whereidea-xx.tar.gz
is the name of the downloaded file. - Navigate to the
bin
directory in the unpacked folder:cd idea-IC-xx/bin
, whereidea-IC-xx
is the name of the unpacked folder. - Run the
idea.sh
script to start IntelliJ IDEA:./idea.sh
- Unpack the downloaded
Your first Java Program: Run using IntelliJ IDEA for the first time
When you run the software, it will ask you accept terms of user agreement. As you check the box for agreement and click continue, you will see the first window.

Here’s a guide on how to use IntelliJ IDEA for the first time:
Step 1: Create a New Project
- Open IntelliJ IDEA. It will open a window like above.
- Click on
New
Project
- In the New Project window, select
Java
for Language. Make sure the Project SDK is set to the correct version of the JDK you installed. If it’s not, click on the box besides “JDK”, select “Add JDK” and navigate to your JDK installation directory. Provide a name for the project; may be “SkillSeminaryJavaTutorial”. You may or may not also change the Location.
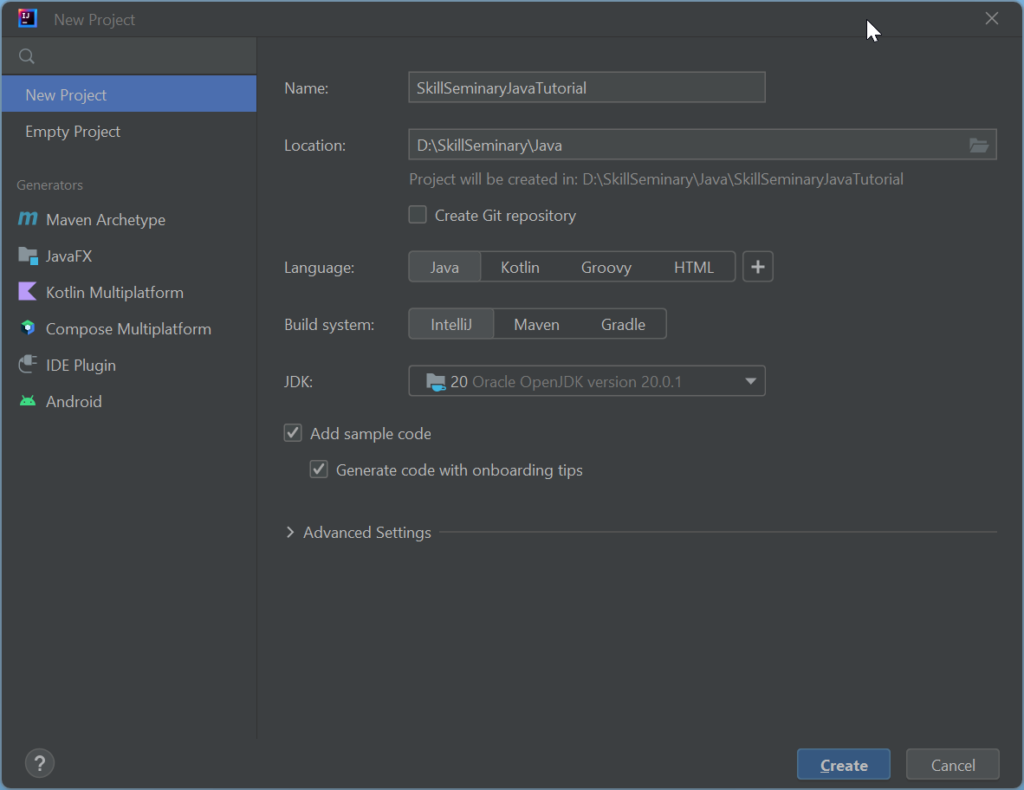
- Click
Create
. This would create a project with sample code.

- You can just run the example code. To run your program, right-click anywhere in the editor window and select
Run 'Main.main()'
. Alternatively, you can click on the green arrow in the toolbar at the top.
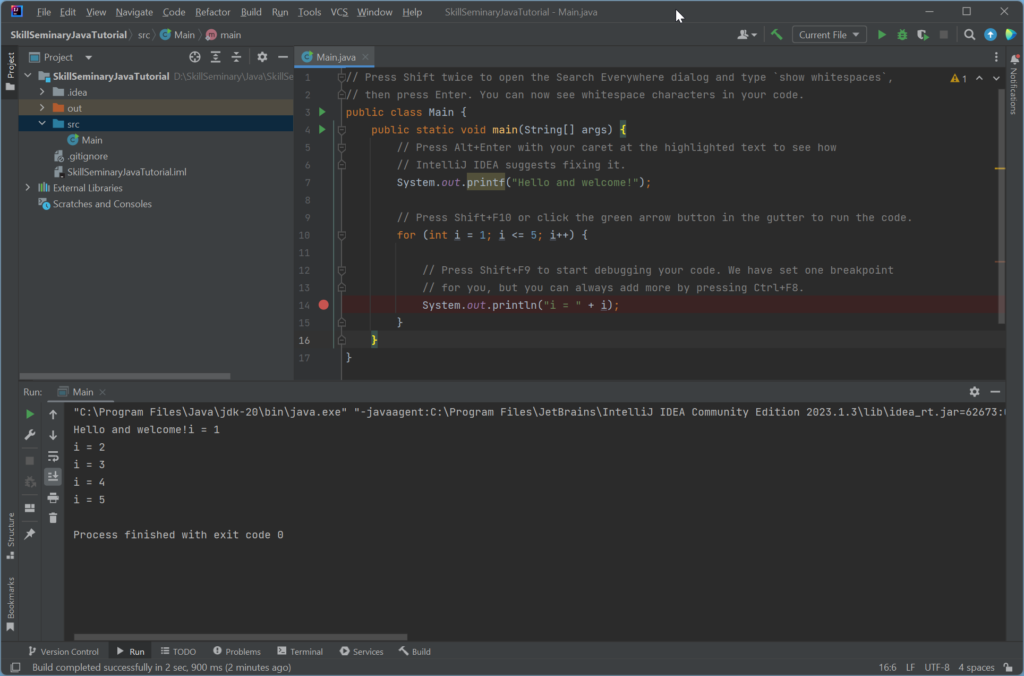
Step 2: Update the main class
- In the Project tool window on the left, select Main inside the
src
folder under your project. Change the code content as follows (then save it):
public class Main {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Java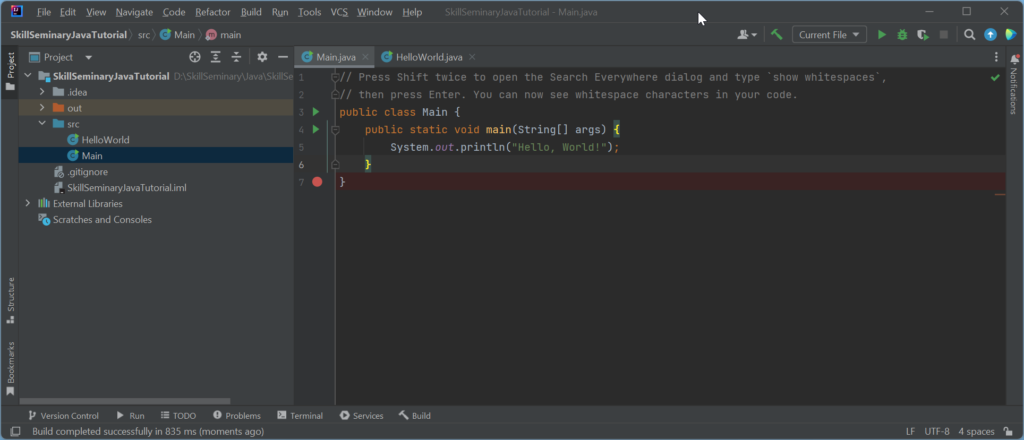
We will explain this code in details in next section. For now, just know that this code will give output “Hello World” on the console.
Step 3: Run the program
- To run your program, right-click anywhere in the editor window and select
Run 'Main.main()'
. Alternatively, you can click on the green arrow in the toolbar at the top. - The output of your program will appear in the Run tool window at the bottom.
Step (optional/alternative): Create a New Java Class instead of updating
- In the Project tool window on the left, right-click on the
src
folder under your project. - Go to
New
>Java Class
. - Give your class a name (for example,
HelloWorld
) and press “Enter”.
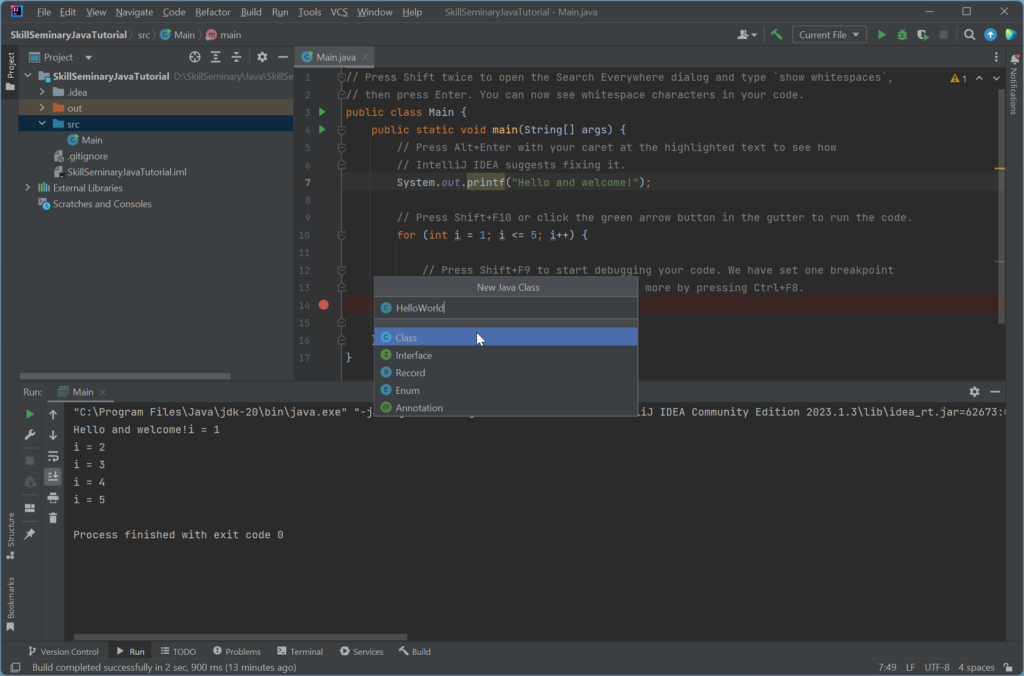
- In the editor window, you can now write your Java code. For example, you could write a simple “Hello, World!” program:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
- To run your program, right-click anywhere in the editor window and select
Run 'HelloWorld.main()'
. Alternatively, you can click on the green arrow in the toolbar at the top. - The output of your program will appear in the Run tool window at the bottom.

Step 4: Save Your Work
IntelliJ IDEA automatically saves your changes every few seconds, so you don’t need to manually save your work. However, if you want to force a save, you can press Ctrl+S
(or Command+S
on macOS).
Step 5: Explore More Features
IntelliJ IDEA has many more features to explore, such as debugging tools, version control integration, and a vast array of plugins to extend its functionality. You can access most of these features through the toolbar at the top or the various tool windows around the edges of the screen.
Remember, the best way to learn is by doing. Don’t be afraid to experiment and try out different features. Happy coding!
Your First Java Program: Let’s understand
Our first Java “Hellow, World” program was as follows:
public class Main {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
JavaShort explanation
Here,
HelloWorld
is a class (wait … but what is a class? Click here to know).main
is a method where the execution of the program begins (again what is a method? Just click here to get an idea about that).- The
System.out.println
statement is used to print output to the console as shown below.

Detailed explanation
Now Let’s break down the “Hello, World!” program in Java:
public class Main { ... }
This is the declaration of a class named Main
. In Java, every application must contain a main class that wraps up all the program code. The keyword public
is an access modifier that means this class can be accessed from anywhere.
public static void main(String[] args) { ... }
This is the main method. Every Java application must have a main
method. It’s the entry point for your application and will subsequently invoke all the other methods required by your program.
public
means that the method is accessible everywhere.static
means the method belongs to theMain
class and not an instance of theMain
class.void
means the method doesn’t return a value.main
is the name of the method.String[] args
is the parameter passed to the main method. It’s an array ofString
objects, allowing you to pass command-line arguments to your program.
System.out.println("Hello, World!");
This is a simple command that prints the text “Hello, World!” to the console.
System
is a built-in class present injava.lang
package. It provides access to system resources.out
is a static member field ofSystem
class and is of typePrintStream
. Its access is allowed to all because it’s a public member.println
is a method ofPrintStream
class. This method is used to print a string followed by a new line.
So, in essence, this program defines a class (Main
), in which the main method resides. The main method then calls System.out.println
to print “Hello, World!” to the console.
Absolutely! Let’s add a section to explain what a class and a method are in Java:
But what is a class and method?
Class
A class in Java is like a blueprint for creating objects. You can think of it as a template or a prototype from which objects are created.
For example, consider a class Car
. A Car
class might specify that all cars have a color, a brand, a model, and a speed. However, the Car
class doesn’t contain any specific information about any individual car.
When you create an object from the Car
class (like Car myCar = new Car();
), that’s when you give the car specific attributes, like its color is red, its brand is Toyota, its model is Corolla, and its speed is 60 mph.
In our “Hello, World!” program, Main
is a class. But we didn’t create any objects from it; we just used it to hold our main
method.
Method
A method in Java is a set of instructions designed to perform a specific task. It’s like a mini-program within your program. You can call it, and it will execute its instructions and then return control back to wherever it was called from.
Methods are useful for organizing your code into logical, manageable chunks. Instead of having all your code in one big block, you can break it down into smaller methods. This makes your code easier to read, test, and debug.
In our “Hello, World!” program, main
is a method. It’s a special method because it’s the entry point for the program. When you run the program, the main
method is called, and it executes its instructions (which is to print “Hello, World!” to the console).
Methods can also take parameters (like String[] args
in the main
method), which are values you can pass into the method when you call it. And methods can return a value back to where they were called from (although the main
method doesn’t return anything, which is why it’s marked with void
).
Wrapping Up
Congratulations! You’ve taken your first steps into the world of Java programming. There’s still a lot to learn, but you’re on the right track. Keep practicing and exploring more features of Java.
Frequently Asked Questions (FAQ)
-
How do I get started with Java?
To get started with Java, you’ll first need to install the Java Development Kit (JDK) on your computer. Then, you can start learning the basics of the Java language, such as variables, data types, operators, control statements, and loops. It’s also a good idea to learn about object-oriented programming (OOP) concepts, as Java is an OOP language.
-
Can I start learning Java as a beginner?
Absolutely! Java is a great language for beginners. It has a straightforward syntax, and it enforces an object-oriented programming model which can help new programmers to understand complex concepts.
-
Is 3 months enough to learn Java?
The time it takes to learn Java can vary widely and depends on several factors, including your prior programming experience, how quickly you learn, and how much time you can dedicate to learning. However, with consistent study and practice, you could gain a solid understanding of the basics of Java in 3 months.
-
Is Java easy or hard for a beginner?
Java is considered one of the easier programming languages to learn, especially if it’s your first language. It has a clear syntax and enforces an object-oriented programming model, which can help new programmers understand complex concepts.
-
What are the best resources to learn Java?
There are many resources available to learn Java, including online tutorials, coding bootcamps, books, and video courses. Some popular options include the official Oracle Java tutorials, Codecademy, Coursera, and Udemy.
-
What are the key concepts to understand in Java?
Some key concepts to understand in Java include variables, data types, operators, control statements, loops, arrays, and object-oriented programming concepts like classes, objects, inheritance, polymorphism, and encapsulation.
-
How can I practice my Java skills?
The best way to practice your Java skills is by writing and running Java programs. Try to solve problems and build projects using Java. Websites like HackerRank, LeetCode, and Codewars offer coding challenges that can help you practice.
-
What are some common mistakes beginners make when learning Java?
Common mistakes include not understanding the difference between Java’s value types and reference types, forgetting to handle exceptions, not properly using Java’s object-oriented features, and writing code without a clear plan or understanding of the problem.
-
How is Java different from other programming languages?
Java is a statically-typed, object-oriented language that’s designed to be platform-independent, meaning that Java programs can run on any device that has a Java Runtime Environment (JRE). This sets it apart from languages that are compiled to machine code specific to a certain processor.
-
What can I build after learning Java?
After learning Java, you can build a wide variety of applications, including desktop applications, web applications, mobile apps (specifically Android apps), and enterprise-scale systems. Java is also commonly used in big data analytics and cloud computing.
Related Tutorials
That’s all for now, folks! Happy coding!