Java do while Loop
Hello there, fellow coder! Today, we’re going to dive into the world of loops in Java, specifically the do-while loop. Ready to loop the loop? Let’s get started!
Introduction
Loops are the bread and butter of any programming language, and Java is no exception. They allow us to perform a set of actions repeatedly, which is pretty handy, right? In this tutorial, we’ll focus on the do-while loop, a special type of loop that guarantees at least one execution of the loop body.
Table of Contents
Understanding the Java do while Loop
So, what’s a do-while loop? It’s a control flow statement that executes a block of code at least once, and then repeatedly executes the block, or not, depending on a given condition at the end of the block. It’s like a while loop, but with a twist!
Syntax of the do while Loop in Java
The syntax of the do-while loop is pretty straightforward:
do {
// Statements
} while(Boolean_expression);
JavaThe Boolean expression appears at the end of the loop, so the statements in the loop execute once before the Boolean is tested. If the Boolean expression is true, the control jumps back up to the do statement, and the statements in the loop execute again. This process repeats until the Boolean expression is false.
Working of the do while Loop
The do-while loop works in a very simple way. It first executes the code block once, then it checks the condition. If the condition is true, it goes back and executes the code block again. This continues until the condition becomes false.
Here’s a flow diagram to help you visualize it:
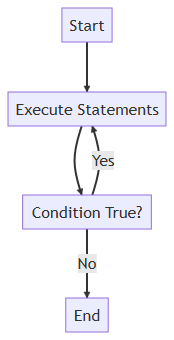
Java do while Loop: Code Examples
Let’s look at some examples to understand the do-while loop better.
Example 1: Simple do-while loop
public class Main {
public static void main(String[] args) {
int i = 1;
do {
System.out.println(i);
i++;
} while(i <= 5);
}
}
JavaIn this example, the loop will print the numbers from 1 to 5. The variable i
is incremented in each iteration. The loop continues until i
is less than or equal to 5.
Example 2: Complex do-while loop
public class Main {
public static void main(String[] args) {
int i = 1, sum = 0;
do {
sum += i;
i++;
} while(i <= 10);
System.out.println("Sum: " + sum);
}
}
JavaIn this example, the loop calculates the sum of the numbers from 1 to 10. The variable sum
holds the total sum, and i
is incremented in each iteration. The loop continues until i
is less than or equal to 10.
Use Cases of the do while Loop
The do-while loop is useful when you need to execute a block of code at least once, regardless of the condition. It’s commonly used when the exact number of iterations is not known in advance, like when reading user input until the user enters a valid input.
Java do-while Loop vs. while Loop
The main difference between the do-while loop and the while loop is that the do-while loop will execute the code block at least once, even if the condition is false, because it checks the condition after executing the block. On the other hand, the while loop will not execute the block if the condition is false at the start.
Let’s illustrate this with some code examples:
Example 1: do-while loop
int i = 5;
do {
System.out.println(i);
i++;
} while(i < 5);
JavaIn this example, even though the condition i < 5
is false at the start, the do-while loop will still execute the code block once. So, the output will be 5
.
Example 2: while loop
int i = 5;
while(i < 5) {
System.out.println(i);
i++;
}
JavaIn this example, the condition i < 5
is false at the start, so the while loop will not execute the code block at all. So, there will be no output.
As you can see, the do-while loop guarantees at least one execution of the loop body, while the while loop does not. This is the key difference between the two.
Wrapping Up
And that’s a wrap on the Java do-while loop! It’s a powerful tool in your Java toolkit, and understanding how it works will help you write more efficient and effective code. Remember, practice makes perfect, so keep coding and have fun!
Frequently Asked Questions (FAQ)
-
What is a Java do-while loop?
A Java do-while loop is a control flow statement that executes a block of code at least once, and then repeatedly executes the block, or not, depending on a given condition at the end of the block.
-
When should you use a do-while loop in Java?
You should use a do-while loop in Java when you need to execute a block of code at least once, regardless of the condition.
-
What is the difference between do and while loop in Java?
The main difference between the do-while loop and the while loop is that the do-while loop will execute the code block at least once, even if the condition is false, because it checks the condition after executing the block. On the other hand, the while loop will not execute the block if the condition is false at the start.
-
How do you use a do-while loop?
You use a do-while loop by writing the keyword
do
, followed by the code block in curly braces{}
, and then the keywordwhile
with the condition in parentheses()
. -
Can a do-while loop be used for array iteration?
Yes, a do-while loop can be used for array iteration, but it’s not common because you need to manually handle the index and check if it’s within the array bounds.
-
Can a do-while loop be nested in Java?
Yes, a do-while loop can be nested in Java. You can have a do-while loop inside another do-while loop.
-
How to break a do-while loop in Java?
You can break a do-while loop in Java using the
break
statement. -
How to continue a do-while loop in Java?
You can continue to the next iteration of a do-while loop in Java using the
continue
statement. -
Can a do-while loop be infinite in Java?
Yes, a do-while loop can be infinite in Java if the condition always evaluates to true.
-
How to handle exceptions in a do-while loop in Java?
You can handle exceptions in a do-while loop in Java using try-catch blocks inside the loop.
Related Tutorials
- Understanding Java while Loop
- Mastering Java for Loop
- Java Control Statements: Break and Continue
- Exception Handling in Java: A Complete Guide
- Java Arrays: A Comprehensive Guide
Happy coding!