Java Exceptions
Hello there, fellow coder! Today, we’re going to dive deep into the world of Java Exceptions. Buckle up, because we’re about to embark on an exciting journey!
Introduction
Ever been in a situation where everything was going smoothly and then, bam! Something unexpected happens. That’s pretty much what a Java Exception is – an unexpected event that disrupts the normal flow of your program. But don’t worry, we’ve got you covered. By the end of this tutorial, you’ll be handling these exceptions like a pro!
Table of Contents
Understanding Java Exceptions
In Java, an Exception is like a hiccup in your program. It’s an event that occurs during the execution of your program and disrupts the normal flow of instructions. But fear not, Java provides us with mechanisms to handle these hiccups and ensure our program can continue to run or fail gracefully.
Types of Java Exceptions
Java Exceptions come in two main flavors: Checked and Unchecked Exceptions.
Checked Exceptions are the polite ones. They occur at compile time and remind you, the programmer, that you need to take care of them. For instance, IOException is a checked exception.
Unchecked Exceptions, on the other hand, are the party crashers. They occur at runtime and are a result of bad programming. NullPointerException, anyone?
Java Exception Hierarchy
In the family tree of Java Exceptions, Throwable is the root. It has two main children:
- Error (for serious stuff that you can’t really handle) and
- Exception (the one we’re interested in).
Exception itself has a child called RuntimeException, which is the parent of all unchecked exceptions.
Java Exception Handling
Exception handling in Java is like having a safety net for your high-wire program. It allows your program to catch and handle errors that occur during execution without crashing. It’s done using the try-catch-finally construct and the throw and throws keywords.
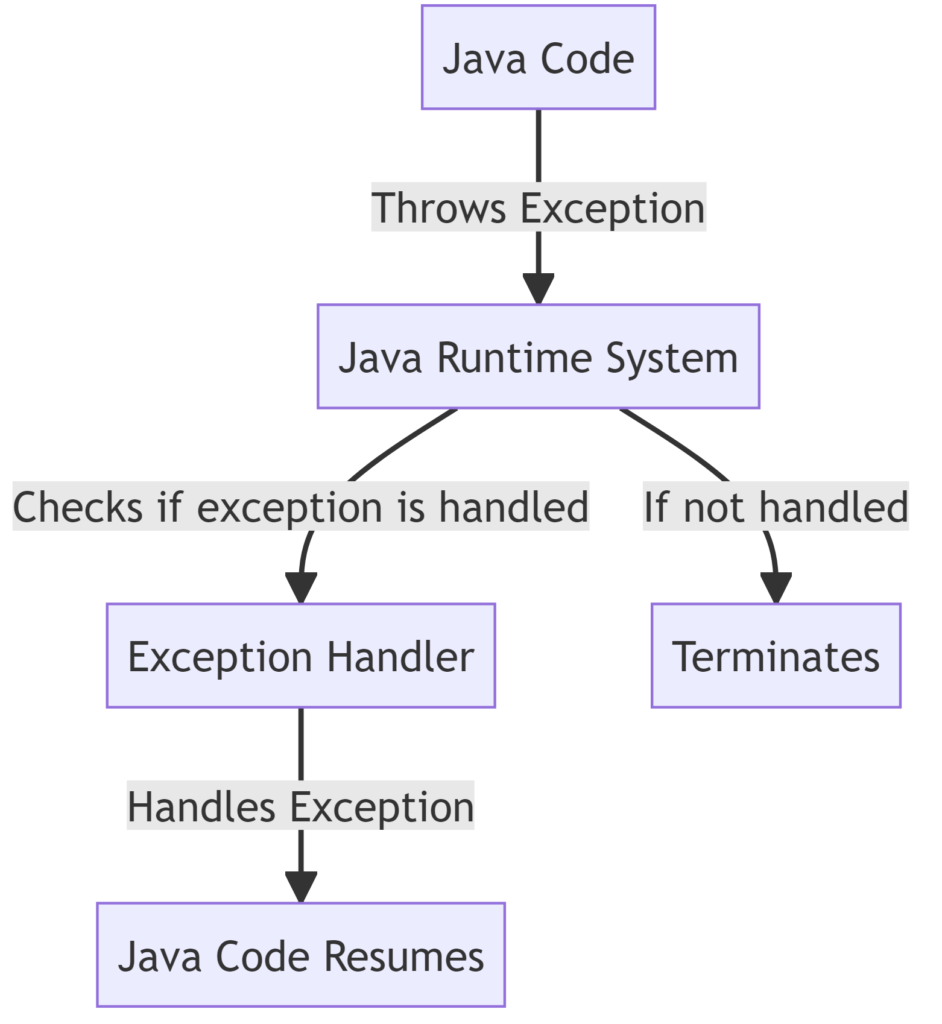
Try-Catch Block in Java
The try-catch block is the bread and butter of exception handling in Java. You put the risky code in the try block, and if an exception occurs, the catch block catches it and decides what to do next.
try {
// Risky code here
} catch (Exception e) {
// Handle exception here
}
JavaExample:
Let’s say we have a piece of code that divides two numbers. But what if the denominator is zero? That would throw an ArithmeticException. Here’s how we can handle it:
try {
int result = 10 / 0; // This will throw an ArithmeticException
} catch (ArithmeticException e) {
System.out.println("You can't divide by zero!");
}
JavaIn this example, the division by zero in the try block throws an ArithmeticException. The catch block catches this exception and prints a friendly error message instead of crashing the program.
The Finally Block in Java
The finally block is like the cleanup crew. It’s a block of code that gets executed no matter what, whether an exception occurs or not. It’s usually used for cleanup tasks like closing a file or a network connection.
try {
// Risky code here
} catch (Exception e) {
// Handle exception here
} finally {
// Cleanup code here
}
JavaExample:
Let’s say we’re reading data from a file. Whether we’re able to read the data successfully or an exception occurs, we want to make sure we close the file afterwards. Here’s how we can do it:
FileReader reader = null;
try {
reader = new FileReader("somefile.txt");
// Read data from the file
} catch (IOException e) {
System.out.println("An error occurred while reading the file.");
} finally {
if (reader != null) {
try {
reader.close();
} catch (IOException e) {
System.out.println("An error occurred while closing the file.");
}
}
}
JavaIn this example, we’re trying to read data from a file in the try block. If an IOException occurs, we catch it and print an error message. Regardless of whether an exception occurred or not, the finally block gets executed and we attempt to close the file. If an exception occurs while closing the file, we catch that as well and print another error message.
Throw Keyword in Java
The throw keyword in Java is used to explicitly throw an exception from a method or any block of code. We can throw either checked or unchecked exceptions.
throw new Exception("This is an exception");
JavaExample:
Let’s say we have a method that sets the age of a person. If the age is negative, we want to throw an IllegalArgumentException.
void setAge(int age) {
if (age < 0) {
throw new IllegalArgumentException("Age cannot be negative");
}
// Set the age
}
JavaIn this example, if the age passed to the setAge method is negative, we throw an IllegalArgumentException with a custom error message.
Throws Keyword in Java
The throws keyword in Java is used to declare that a method might throw a certain exception. It’s usually used for checked exceptions, which must be declared in the method signature if they’re not caught within the method.
void riskyMethod() throws IOException {
// Risky IO operations here
}
JavaExample:
Let’s say we have a method that reads data from a file. This method might throw an IOException, so we declare it using the throws keyword.
void readFile(String filename) throws IOException {
FileReader reader = new FileReader(filename);
// Read data from the file
}
JavaIn this example, the readFile method declares that it might throw an IOException. Any code that calls this method will have to handle this exception.
Custom Exceptions in Java
In Java, we can create our own custom exceptions by extending the Exception class. Custom exceptions are useful for representing specific error conditions within your application.
class CustomException extends Exception {
public CustomException(String message) {
super(message);
}
}
JavaExample:
Let’s say we’re writing a banking application, and we want to throw a custom exception when a withdrawal would result in a negative balance.
class InsufficientFundsException extends Exception {
public InsufficientFundsException(String message) {
super(message);
}
}
void withdraw(double amount) throws InsufficientFundsException {
if (amount > balance) {
throw new InsufficientFundsException("Insufficient funds for this withdrawal");
}
// Perform the withdrawal
}
JavaIn this example, we’ve created a custom exception called InsufficientFundsException. In the withdraw method, if the withdrawal amount is greater than the balance, we throw this custom exception.
Best Practices for Exception Handling in Java
When it comes to exception handling in Java, there are a few best practices to keep in mind:
- Don’t swallow exceptions: Always at least log the exception so that you can debug it later.
- Throw specific exceptions: Instead of throwing the general Exception class, throw a specific exception like IllegalArgumentException or IOException.
- Don’t catch Throwable or Error: These are serious problems that your application should not attempt to handle.
Code Examples
Let’s look at a couple of code examples to see how exception handling works in Java.
Example 1: Handling an ArrayIndexOutOfBoundsException
try {
int[] numbers = {1, 2, 3};
System.out.println(numbers[3]); // This will throw an exception
} catch (ArrayIndexOutOfBoundsException e) {
System.out.println("Oops! You tried to access an index that doesn't exist.");
}
JavaIn this example, we’re trying to access the fourth element of an array that only has three elements. This will throw an ArrayIndexOutOfBoundsException. But since we’ve wrapped the risky code in a try block and provided a catch block to handle the exception, instead of crashing, our program will simply print out a friendly error message.
Example 2: Creating and Using a Custom Exception
// Creating a custom exception
class CustomException extends Exception {
public CustomException(String message) {
super(message);
}
}
// Using the custom exception
try {
throw new CustomException("This is a custom exception!");
} catch (CustomException e) {
System.out.println(e.getMessage());
}
JavaIn this example, we’re creating our own custom exception by extending the Exception class. We can then throw and catch this custom exception just like any other exception. When we catch the custom exception, we print out the custom message that we provided when we threw the exception.
Wrapping Up
Well, that’s a wrap! We’ve covered a lot of ground in this tutorial, from understanding what Java Exceptions are, to handling them using try-catch blocks, and even creating our own custom exceptions. Remember, exception handling is your friend – it helps you build robust and fault-tolerant programs.
Frequently Asked Questions (FAQ)
-
What are the 3 Java exceptions?
In Java, exceptions are divided into three types: checked exceptions, unchecked exceptions, and errors. Checked exceptions are exceptions that need to be declared in a method or constructor’s throws clause if they can be thrown by the execution of the method or constructor and propagate outside the method or constructor boundary. Unchecked exceptions are exceptions that do not need to be declared in a method or constructor’s throws clause. Errors are exceptional conditions that are external to the application, and that the application usually cannot anticipate or recover from.
-
What are the Java exceptions?
Java exceptions are events that disrupt the normal flow of the program. They are objects that are thrown out of the methods and need to be caught and handled by other parts of the program.
-
What are the 4 checked exceptions in Java?
Some examples of checked exceptions in Java include IOException, SQLException, ClassNotFoundException, and InvocationTargetException. These are exceptions that need to be declared in a method or constructor’s throws clause if they can be thrown by the execution of the method or constructor.
-
What are the 5 exception keywords in Java?
The five keywords used in handling exceptions in Java are try, catch, finally, throw, and throws.
-
How does the try-catch block work in Java?
The try block encloses the code that might throw an exception, while the catch block contains the code that handles the exception. If an exception occurs in the try block, the flow of control transfers to the appropriate catch block where the exception is handled.
-
What is the difference between checked and unchecked exceptions?
Checked exceptions are exceptions that are checked at compile time. If some code within a method throws a checked exception, then the method must either handle the exception or it must specify the exception using the throws keyword. Unchecked exceptions are exceptions that are checked at runtime. These are also called as Runtime Exceptions.
-
What is the Java Exception hierarchy?
The base class of all exceptions is java.lang.Throwable. It has two child objects – Error and Exception. Errors are exceptional scenarios that are out of scope of application and it’s not possible to foresee and recover from them, for example hardware failure, JVM crash or out of memory error. Exceptions are further divided into checked exceptions and runtime exception.
-
How do I create a custom exception in Java?
You can create a custom exception in Java by extending the Exception class. In your new class, you can add custom fields and methods. You can create a constructor for your exception class that accepts a message parameter and passes it to the constructor of the superclass.
-
What are some best practices for exception handling in Java?
Some best practices for exception handling in Java include: don’t ignore exceptions, don’t catch the Exception class (catch more specific exceptions instead), don’t throw Throwable or Error, always clean up after yourself in a finally block, and use custom exceptions for your application’s specific needs.
-
What is the finally block in Java?
The finally block in Java is a block that follows a try-catch block. It is always executed whether an exception is handled or not. This makes it a good place to put cleanup code that should run regardless of what happens in the try-catch block.
Related Tutorials
- Java Multithreading: Learn how to write Java programs that do many things at once.
- Java I/O: Get a handle on Java’s powerful I/O libraries for reading and writing data.
- Java Collections: Master Java’s built-in data structures for storing and organizing data.
And that’s it, folks! I hope you found this tutorial helpful. Remember, practice makes perfect, so keep coding and have fun!