JavaScript Interactions: Alert, Prompt, and Confirm
Introduction
Hello there, JavaScript enthusiasts! Today, we’re going to dive into the world of JavaScript interactions. We’ll be focusing on three key players: alert
, prompt
, and confirm
. These are the bread and butter of user interactions in JavaScript, and mastering them is like getting a backstage pass to the JavaScript concert. So, are you ready to rock and roll? Let’s get started!
Table of Contents
JavaScript Alert
First up, we have the alert
function. This is like the drummer of our JavaScript band – it sets the beat and gets everyone’s attention.
alert("Hello, World!");
JavaScriptThis line of code will pop up a box with the message “Hello, World!”. Simple, right? But what if we want to customize our alert? Well, that’s a bit like asking the drummer to change the beat. It’s not built into the basic function, but with a bit of creativity (and a lot of Googling), you can find ways to make it happen.
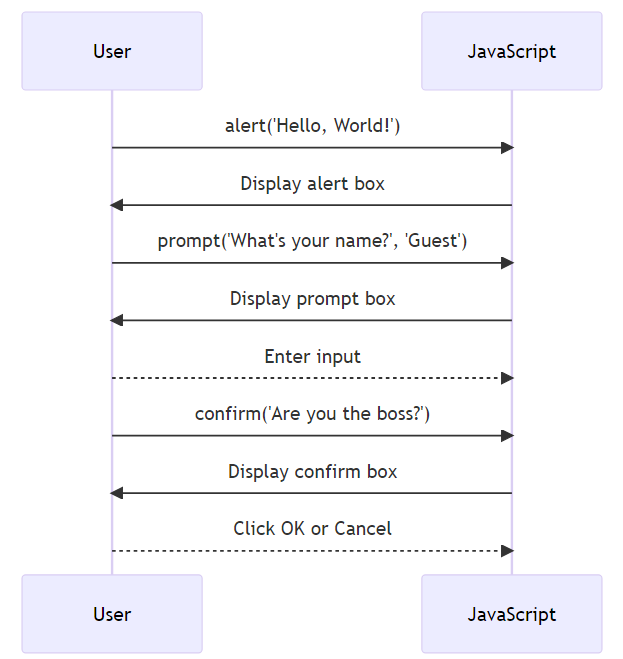
JavaScript Prompt
Next, we have the prompt
function. This is like the lead singer – it’s the one that interacts most directly with the user.
let name = prompt("What's your name?", "Guest");
JavaScriptThis code will open a dialog box asking the user “What’s your name?”. If the user doesn’t enter anything, the default value will be “Guest”. The value entered by the user will be stored in the name
variable.
JavaScript Confirm
Finally, we have the confirm
function. This is like the guitarist – it plays a key role in deciding the direction of the song (or in this case, the program).
let isBoss = confirm("Are you the boss?");
JavaScriptThis line of code will open a dialog box with the question “Are you the boss?”. If the user clicks “OK”, the function will return true
, and if they click “Cancel”, it will return false
.
JavaScript Interactions with HTML and CSS
Now that we’ve met the band, let’s see them in action. JavaScript can interact with HTML and CSS to create dynamic web pages. For example, you can use JavaScript to change the content of an HTML element:
document.getElementById("welcome").innerHTML = "Hello, JavaScript!";
JavaScriptOr to change the style of an element:
document.getElementById("welcome").style.fontSize = "25px";
JavaScriptCode Examples
Let’s put it all together with a couple of code examples.
- Code Example 1: Custom Greeting
let name = prompt("What's your name?", "Guest");
alert("Hello, " + name + "!");
JavaScriptThis code will ask the user for their name, and then greet them with an alert. If the user doesn’t enter a name, they will be greeted as “Guest”.
- Code Example 2: Age Verification
let isOver18 = confirm("Are you over 18?");
if (isOver18) {
alert("Welcome to our website!");
} else {
alert("Sorry, you must be over 18 to visit our website.");
}
JavaScriptThis code will ask the user if they are over 18. If they click “OK”, they will be welcomed to the website. If they click “Cancel”, they will be told that they must be over 18 to visit the website.
Wrapping Up
And that’s a wrap! We’ve covered the basics of JavaScript interactions using alert
, prompt
, and confirm
. With these tools in your toolkit, you’re
well on your way to becoming a JavaScript rockstar. Remember, practice makes perfect, so don’t be afraid to play around with these functions and see what you can create.
Frequently Asked Questions (FAQ)
-
What is the purpose of the
alert
function in JavaScript?The
alert
function is used to display a dialog box with a specified message and an OK button. It’s a way to provide information to the user and doesn’t return any value. -
How can I get user input in JavaScript?
The
prompt
function is used to get user input in JavaScript. It displays a dialog box with a specified message and an input field, and returns the input value as a string. -
What does the
confirm
function return in JavaScript?The
confirm
function displays a dialog box with a specified message and two buttons, OK and Cancel. It returnstrue
if the user clicks OK andfalse
if the user clicks Cancel. -
Can I customize the look of JavaScript dialog boxes?
The look of
alert
,prompt
, andconfirm
dialog boxes is determined by the browser and cannot be customized with JavaScript. However, you can create custom dialog boxes using HTML, CSS, and JavaScript. -
How does JavaScript interact with HTML and CSS?
JavaScript can interact with HTML and CSS through the Document Object Model (DOM). It can be used to change the content and style of HTML elements, respond to user events, and create dynamic web pages.
-
Can I use JavaScript to change the content of an HTML element?
Yes, you can use the
innerHTML
property to change the content of an HTML element. For example,document.getElementById("demo").innerHTML = "Hello, JavaScript!";
-
Can I use JavaScript to change the style of an HTML element?
Yes, you can use the
style
property to change the style of an HTML element. For example,document.getElementById("demo").style.fontSize = "25px";
-
How can I store the value returned by the
prompt
function in a variable?You can store the value returned by the
prompt
function in a variable like this:let name = prompt("What's your name?", "Guest");
-
What happens if the user clicks “Cancel” in a
prompt
dialog box?If the user clicks “Cancel” in a
prompt
dialog box, the function returnsnull
. -
What happens if the user clicks “Cancel” in a
confirm
dialog box?If the user clicks “Cancel” in a
confirm
dialog box, the function returnsfalse
.
Related Tutorials
- Introduction to JavaScript
- Getting Started
- JavaScript Syntax: A Fun, Free and Easy Tutorial
- JavaScript Comments
- JavaScript Variables
- JavaScript var Explained
- JavaScript Let
- JavaScript const
- JavaScript Data Types
And there you have it, folks! A comprehensive, step-by-step guide to JavaScript interactions. Remember, the key to mastering JavaScript (or any programming language, for that matter) is practice. So go ahead, roll up your sleeves, and start coding!