Mastering JavaScript Events
Hello, budding web developers! Today, we’re diving into the world of JavaScript Events. Whether you’re a newbie or a seasoned coder, this guide will help you understand and master JavaScript events. So, let’s get started!
Table of Contents
Introduction to JavaScript Events
JavaScript events are the bread and butter of interactive web development. They’re the magic that brings your web pages to life, responding to user actions like clicks, key presses, and mouse movements. But what exactly are they? Let’s find out!
Understanding the Event Model
In JavaScript, an event is a signal that something has happened. This could be a user action (like a click or a keypress), a browser action (like a page load), or even a programmatic action (like an AJAX response).
When these events occur, we can use JavaScript to respond to them – this is where event handlers and listeners come into play. They’re like the vigilant sentinels of your code, always on the lookout for specific events to occur.
And here is a sequence diagram that illustrates the flow of JavaScript events:
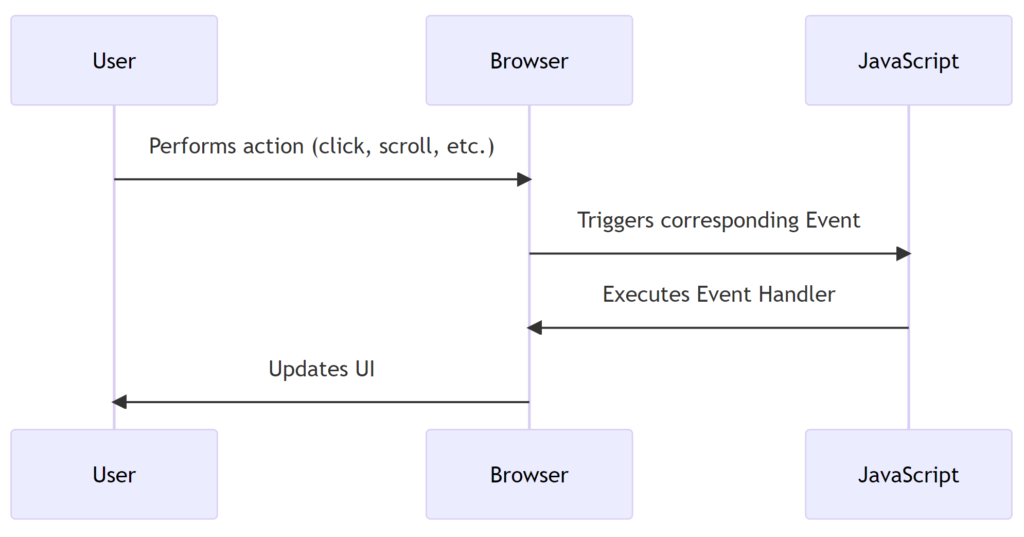
Working with JavaScript Events
So, how do we work with these events? It’s simple – we attach an event handler to an event. This handler is a block of code (usually a function) that runs when the event occurs. Let’s look at a simple example:
// Here's our event handler
function sayHello() {
alert('Hello, world!');
}
// And here's how we attach it to an event
document.querySelector('button').addEventListener('click', sayHello);
In this example, whenever the button is clicked, the sayHello
function runs, and a friendly greeting pops up. Easy, right?
JavaScript Event Types
There are many types of events in JavaScript, each corresponding to different user actions. Here are a few key ones:
click
: Fires when a mouse button is clicked.keydown
: Fires when a key is pressed down.load
: Fires when a page has fully loaded.
Each of these events can be listened for and handled using the techniques we’ve discussed.
Code Examples
Let’s look at a couple of practical examples to see these events in action.
// Example 1: Responding to a click event
document.querySelector('button').addEventListener('click', function() {
alert('Button clicked!');
});
// Example 2: Responding to a page load event
window.addEventListener('load', function() {
console.log('Page fully loaded and ready to go!');
});
In the first example, an alert pops up whenever the button is clicked. In the second example, a message is logged to the console when the page finishes loading.
Advanced Topics
As you delve deeper into JavaScript events, you’ll encounter concepts like event propagation (bubbling and capturing) and event delegation. These are advanced topics that can give you greater control over how events are handled in your code.
Wrapping Up
JavaScript events are a crucial part of interactive web development. By understanding and mastering events, you can create dynamic, responsive web pages that provide a great user experience.
Frequently Asked Questions (FAQ)
What are the different types of JavaScript events?
JavaScript supports a wide range of events. Some of the most common ones include:
1) click
: Triggered when a user clicks on an element.
2) mouseover
: Triggered when a user moves the mouse over an element.
3) mouseout
: Triggered when a user moves the mouse away from an element.
4) keydown
: Triggered when a user presses a key.
5) load
: Triggered when a page or an image is finished loading.
How do I declare an event in JavaScript?
In JavaScript, you don’t declare events. Instead, you listen for them. You can do this using the addEventListener
method. Here’s an example:
document.querySelector('button').addEventListener('click', function() {
console.log('Button clicked!');
});
JavaScriptIn this example, we’re listening for a click
event on a button. When the button is clicked, the function is executed.
What is event propagation?
Event propagation is the process by which an event triggers its handlers on an element and its ancestors (parent, grandparent, etc.). There are two types of event propagation: bubbling and capturing.
1) Bubbling: The event is first captured and handled by the innermost element and then propagated to outer elements.
2) Capturing: The event is first captured by the outermost element and propagated to the inner elements.
How does event delegation work?
Event delegation is a technique in which you delegate the handling of an event to a parent element. This is particularly useful when you have a lot of elements that should behave in the same way when an event occurs. Instead of adding an event listener to each element individually, you add one listener to the parent element.
Can I remove an event handler once it’s been added?
Yes, you can remove an event handler using the removeEventListener
method. You need to pass the same event and function to removeEventListener
as you did to addEventListener
. Here’s an example:
function handleClick() {
console.log('Button clicked!');
}
let button = document.querySelector('button');
button.addEventListener('click', handleClick);
// Later in your code...
button.removeEventListener('click', handleClick);
JavaScriptIn this example, the handleClick
function will no longer be executed when the button is clicked.
Related Tutorials
- JavaScript Operators
- JavaScript Arithmetic Operators
- JavaScript Logical Operators
- JavaScript Comparison Operators
- JavaScript Type Conversion
- JavaScript Control Structures
- JavaScript Loops
- JavaScript Functions
And that’s a wrap! We hope you found this guide helpful. Keep coding, and stay curious!