CSS Syntax and Selectors
Welcome to our comprehensive guide on CSS syntax and selectors! In this tutorial, we will explore the fundamental aspects of CSS, from its syntax to the various types of selectors available. Whether you’re a beginner looking to understand the basics or an experienced developer seeking a refresher, this tutorial has got you covered. Let’s dive in!
Table of Contents
Introduction to CSS
CSS, short for Cascading Style Sheets, is a powerful language used to style the visual presentation of web pages. It allows developers to control the layout, colors, fonts, and other visual aspects of HTML elements. By separating the content (HTML) from the presentation (CSS), CSS provides flexibility and maintainability to web designs.
CSS Syntax
Before we dive into the various CSS selectors, let’s familiarize ourselves with the syntax of CSS rules. CSS rules consist of a selector and one or more declarations. The selector determines which elements the rule applies to, while the declarations define the styling properties and their values.
selector {
property: value;
/* additional properties */
}
CSSIn the example above, the selector targets elements to be styled, and the properties specify the desired styling aspects. For instance, to set the color of all paragraphs to red, we can use the following CSS rule:
p {
color: red;
}
CSSOutput
If you run the above code online Clicking the “Run this Code Online” button, you will see how the above CSS code is styling above HTML.
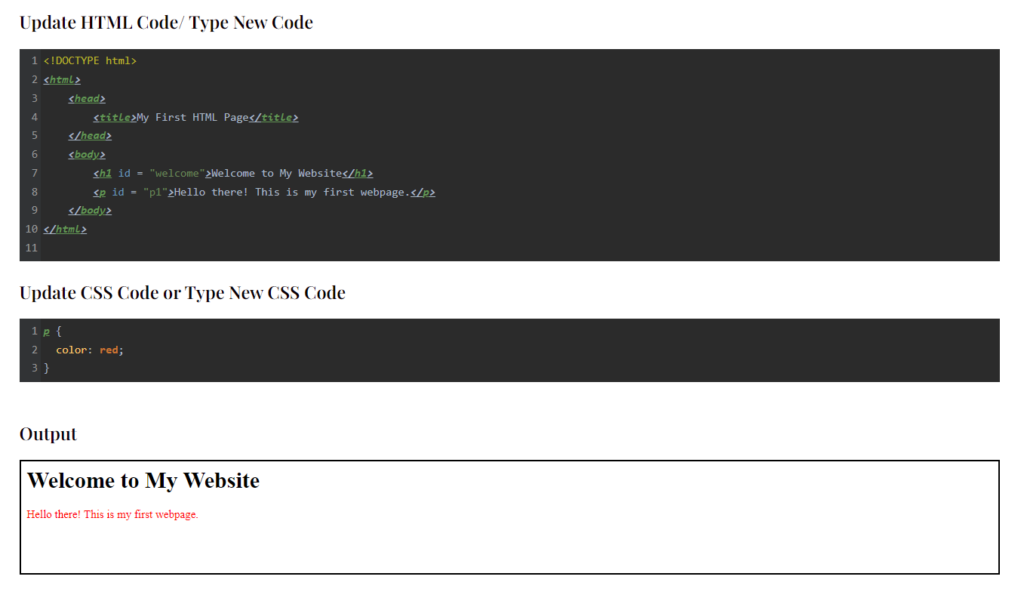
CSS Selectors
CSS selectors are used to target specific HTML elements for styling. They allow you to select elements based on various criteria, such as their ID, class, tag name, or attributes. Let’s explore the most commonly used selectors.
ID Selectors
An ID selector targets a specific element based on its unique ID attribute. To select an element by its ID, prefix the ID name with a hash (#) symbol. For example:
#my-element {
/* CSS declarations */
}
CSSClass Selectors
Class selectors are used to select elements that share the same class attribute. To select elements by class, prefix the class name with a dot (.) symbol. For example:
.my-class {
/* CSS declarations */
}
CSSElement Selectors
Element selectors target elements based on their tag name. They select all occurrences of a specific HTML element. For example:
h1 {
/* CSS declarations */
}
CSSUniversal Selectors
The universal selector, denoted by an asterisk (*), matches any element in the document. It can be useful for applying styles globally or resetting default styles. For example:
* {
/* CSS declarations */
}
CSSAttribute Selectors
Attribute selectors allow you to select elements based on their attributes and attribute values. They offer more precise targeting options. For example:
input[type="text"] {
/* CSS declarations */
}
CSSPseudo-Class and Pseudo-Element Selectors
Pseudo-classes and pseudo-elements target elements based on their state or position within the document. They provide additional styling options and interactivity. Examples include :hover
, :active
, :before
, and :after
. For example:
a:hover {
/* CSS declarations */
}
CSSCheck out our CSS Pseudo-classes and Pseudo-elements tutorials for more info!
Combining Selectors
CSS allows you to combine multiple selectors to target specific elements. This technique is known as selector combination or chaining. It provides powerful control over styling elements based on complex criteria. For example:
ul.nav li {
/* CSS declarations */
}
CSSSpecificity
Specificity is an important concept in CSS that determines which styles take precedence when multiple rules target the same element. Understanding specificity helps avoid conflicts and ensures the desired styles are applied. Specificity is calculated based on the types of selectors used. The more specific a selector, the higher its priority. For example:
/* Specificity: ID > Class > Element */
#my-element {
color: red;
}
CSSInheritance
In CSS, inheritance allows certain properties to be automatically passed down from parent elements to their children. This can reduce the need for repetitive styling and make your stylesheets more concise. Understanding how inheritance works is crucial for efficient CSS development. For example:
.parent {
color: blue;
}
.child {
/* Inherits color: blue from parent */
}
CSSCode Examples
Now, let’s explore some code examples to solidify our understanding of CSS syntax and selectors.
/* Example 1: Styling paragraphs with a class */
.my-class {
font-size: 16px;
color: #333;
}
/* Example 2: Selecting elements by attribute */
input[type="submit"] {
background-color: #007bff;
color: #fff;
}
/* Example 3: Using pseudo-classes */
a:hover {
text-decoration: underline;
}
/* Example 4: Combining selectors */
ul.nav li {
list-style: none;
}
/* Example 5: Specificity in action */
#my-element {
color: red;
}
CSSWrapping Up
Congratulations! You’ve now learned the essentials of CSS syntax and selectors. You understand how to target specific elements, apply styles, and control specificity and inheritance. With this knowledge, you can create visually appealing and well-structured web designs. Remember to experiment and practice with different selectors to unleash the full potential of CSS.
Frequently Asked Questions
What is CSS syntax?
CSS syntax refers to the structure and rules for writing CSS code. It consists of selectors and declarations, where selectors target elements and declarations define the styling properties and their values.
How do ID selectors work in CSS?
ID selectors in CSS are used to target elements based on their unique ID attributes. To select an element by its ID, prefix the ID name with a hash (#) symbol.
Can I select multiple elements with the same class using CSS?
Yes, you can select multiple elements with the same class using class selectors in CSS. Prefix the class name with a dot (.) symbol to target elements sharing that class.
What are pseudo-classes and pseudo-elements in CSS?
Pseudo-classes and pseudo-elements are CSS selectors that target elements based on their state or position. Pseudo-classes, such as :hover
or :active
, select elements based on user interactions, while pseudo-elements, such as :before
or :after
, create virtual elements for additional styling. Check out CSS Pseudo-classes and Pseudo-elements for more info
How does specificity affect CSS styles?
Specificity determines which styles take precedence when multiple rules target the same element. The more specific a selector, the higher its priority in overriding conflicting styles.
Can I combine multiple selectors in CSS?
Yes, you can combine multiple selectors in CSS to target specific elements. This technique, known as selector combination or chaining, provides powerful control over styling elements based on complex criteria.
Are CSS styles inherited by default?
Some CSS properties are inherited by default, meaning they are automatically passed down from parent elements to their children. However, not all properties inherit their values, and some may require explicit styling.
How can I calculate the specificity of CSS selectors?
Specificity is calculated based on the types of selectors used. Each selector has a specific weight: ID selectors have the highest weight, followed by class selectors, element selectors, and universal selectors. The more specific the selector, the higher its weight.
Can I override inherited styles in CSS?
Yes, you can override inherited styles in CSS by applying more specific styles to the desired elements. By increasing the specificity of your selectors, you can ensure that the desired styles take precedence over inherited styles.
What CSS properties do not inherit by default?
Some common CSS properties that do not inherit by default include border
, padding
, margin
, width
, height
, background
, and display
. These properties require explicit styling for each element.
Related Tutorials
Here are some related tutorials you may find helpful:
- Introduction to HTML: Learn the basics of HTML and how to structure web pages.
- CSS Box Model Explained: Dive deeper into the CSS box model and its impact on layout.
- CSS Flexbox: Discover powerful CSS layout techniques using flexbox.
- Responsive Web Design with CSS Media Queries – Learn how to create responsive websites that adapt to different screen sizes.
Happy coding and styling!