Ah, WordPress! Remember the days when it was just for blogging? Neither do we! With the evolution of WordPress, incorporating JavaScript has become a common practice to enhance the user experience. Let’s dive into the nitty-gritty of how to add that magical touch of JavaScript to your WordPress page.
Table of Contents
Why Add JavaScript to WordPress?
JavaScript is not just a sprinkle of magic dust; it’s a powerful tool that:
- Enhances User Experience: From smooth animations to interactive forms, JavaScript can make your site more engaging.
- Adds Interactive Elements: Sliders, pop-ups, and dynamic content are all made possible with JavaScript.
- Integrates Third-party Applications: Want to add a chatbot or a dynamic weather widget? JavaScript’s got you covered.
Understanding the Basics
Before we jump into the deep end, let’s get our basics right:
- Inline vs. External JavaScript: Inline scripts are part of your HTML document, while external scripts are separate files. While inline scripts can be quick to implement, external scripts are more organized and cacheable.
- JavaScript Dependencies in WordPress: Some scripts rely on others to function correctly. For instance, a script that uses jQuery functions will need jQuery to be loaded first. WordPress has a built-in system to handle this, ensuring scripts load in the correct order.
Diagram: Here’s a flowchart showing how JavaScript interacts with WordPress elements.
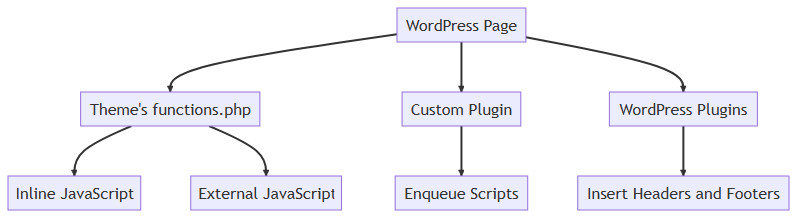
Methods to Add JavaScript to WordPress
Using the Theme’s Functions.php File
This method involves adding your script via the theme’s functions.php
file. The functions.php
file acts as a theme’s plugin, allowing you to modify WordPress functionality.
Steps:
- Navigate to your WordPress dashboard.
- Go to
Appearance
>Theme Editor
. - Find the
functions.php
file in the right sidebar. - Add your JavaScript code or link to an external JavaScript file.
Example:
function add_my_script() {
wp_enqueue_script('my-script', get_template_directory_uri() . '/js/my-script.js', array('jquery'), '1.0', true);
}
add_action('wp_enqueue_scripts', 'add_my_script');
PHPThis code enqueues a script named my-script
located in the theme’s js
directory.
Pros:
- Quick and straightforward for theme-specific functionalities.
- No need for additional plugins.
Cons:
- If you change or update your theme, you might lose the script.
- Not ideal for functionalities you want to keep across different themes.
Using a Custom Plugin
Creating a custom plugin to enqueue scripts ensures that your script stays, no matter which theme you use. This method is more advanced but offers greater flexibility.
Steps:
- Navigate to your WordPress directory using an FTP client or cPanel.
- Go to
wp-content
>plugins
. - Create a new folder for your custom plugin, e.g.,
my-custom-plugin
. - Inside this folder, create a PHP file, e.g.,
my-custom-plugin.php
. - Add your plugin details and JavaScript code or link to the file.
Example:
<?php
/*
Plugin Name: My Custom Plugin
Description: A plugin to add custom JavaScript.
Version: 1.0
Author: Your Name
*/
function add_my_script_plugin() {
wp_enqueue_script('my-script-plugin', plugin_dir_url( __FILE__ ) . 'js/my-script-plugin.js');
}
add_action('wp_enqueue_scripts', 'add_my_script_plugin');
PHPThis code enqueues a script located in the js
directory of the plugin.
Pros:
- Scripts remain intact regardless of theme changes.
- Offers better organization for larger functionalities.
Cons:
- Requires a bit more setup than the
functions.php
method. - Might be overkill for small script additions.
Using WordPress Plugins
For those who aren’t comfortable with coding or want a more user-friendly approach, there are several WordPress plugins designed to help you add custom scripts.
Steps:
- Navigate to your WordPress dashboard.
- Go to
Plugins
>Add New
. - Search for a plugin like “Insert Headers and Footers” or “Simple Custom CSS and JS”.
- Install and activate the chosen plugin.
- Follow the plugin’s instructions to add your JavaScript.
Example:
Using the “Insert Headers and Footers” plugin:
- After activation, go to
Settings
>Insert Headers and Footers
. - Paste your JavaScript code or link in the appropriate section.
- Save changes.
Pros:
- User-friendly, especially for those not familiar with coding.
- Provides a GUI to manage scripts.
Cons:
- Reliance on third-party plugins which might not always be updated.
- Some plugins might add unnecessary bloat to your website.
By understanding the pros and cons of each method, you can choose the one that best suits your needs and expertise level. Whether you’re a coding novice or a seasoned developer, WordPress offers a flexible platform to integrate JavaScript and enhance your website’s functionality.
Footers” that make the process even simpler. Just install, paste your script, and voila! This is perfect for those who aren’t comfortable with coding but still want to add custom scripts.
Methods to Add JavaScript on a Single WordPress Page or Post
Sometimes, you might want to run a JavaScript only on a specific page or post rather than the entire website. This can be useful for scripts tailored to unique content or functionalities. Here’s how you can achieve this:
Using Conditional Tags with functions.php
Steps:
- Navigate to your WordPress dashboard.
- Go to
Appearance
>Theme Editor
. - Find the
functions.php
file in the right sidebar. - Use WordPress conditional tags to target a specific page or post.
Example:
function add_my_single_page_script() {
if (is_page('sample-page')) { // Replace 'sample-page' with your page slug
wp_enqueue_script('my-single-page-script', get_template_directory_uri() . '/js/my-single-page-script.js', array('jquery'), '1.0', true);
}
}
add_action('wp_enqueue_scripts', 'add_my_single_page_script');
PHPThis code checks if the current page is “sample-page” and then enqueues the script. You can replace 'sample-page'
with the slug of your desired page.
For a specific post, you can use:
if (is_single('post-id')) { ... }
PHPReplace 'post-id'
with the ID of your post.
Pros:
- Allows for targeted script execution.
- Efficient, as the script only loads where needed.
Cons:
- Requires manual intervention if you want to target multiple pages or posts.
- If you change or update your theme, you might lose the script.
Using a Plugin: Conditional Script Loading
For those who prefer a GUI approach, there are plugins like “Conditional Script Loading” that allow you to load scripts based on specific conditions.
Steps:
- Navigate to your WordPress dashboard.
- Go to
Plugins
>Add New
. - Search for “Conditional Script Loading” or a similar plugin.
- Install and activate the chosen plugin.
- Follow the plugin’s instructions to set conditions for your JavaScript.
Pros:
- User-friendly interface.
- Provides more flexibility with conditions, such as targeting categories, tags, or custom post types.
Cons:
- Reliance on third-party plugins.
- Might add some overhead to your website.
By using either of these methods, you can ensure that your JavaScript only runs on the pages or posts you specify, optimizing performance and ensuring a tailored user experience.
Best Practices
- Ensure Compatibility: Always test your scripts to ensure they don’t conflict with other plugins or themes.
- Optimize for Performance: Minify and compress scripts for faster load times. Tools like JSCompress can help.
- Prioritize User Experience: Always keep the user in mind. Ensure your scripts enhance the site without compromising speed or usability.
Common Mistakes to Avoid
- Hardcoding Scripts: Avoid adding scripts directly into header or footer templates. This can lead to issues when updating themes or plugins.
- Ignoring Dependencies: Always ensure your scripts load in the correct order, especially if they rely on libraries like jQuery.
- Overloading with Scripts: While it’s tempting to add every cool feature you find, too many scripts can slow down your site. Be selective!
JavaScript Examples
Adding an Interactive Button
document.getElementById("myBtn").addEventListener("click", function() {
alert("Hello World!");
});
JavaScriptThis simple code adds an alert when a button with the ID “myBtn” is clicked. It’s a basic example of how you can make your site interactive with just a few lines of JavaScript.
Integrating a Third-party API
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data));
JavaScriptThis code fetches data from a third-party API and logs it to the console. It’s a starting point for integrating external data sources into your WordPress site.
Conclusion
Incorporating JavaScript into your WordPress site can seem daunting at first, but with the right knowledge and tools, it becomes a breeze. Remember, the digital world is ever-evolving. Stay curious, keep experimenting, and always aim to enhance the user experience. Happy coding! 🚀
Frequently Asked Questions
Why is my JavaScript not working in WordPress?
This could be due to a variety of reasons, including script conflicts, incorrect placement of the script, or caching issues. Always ensure your scripts are enqueued correctly and clear your cache after adding new scripts.
Can I add JavaScript to WordPress without a plugin?
Absolutely! You can add JavaScript directly to your theme’s functions.php
file or through a custom plugin. However, using a plugin can make managing scripts easier.
How do I test if my JavaScript is running correctly?
Open your website and right-click to select “Inspect” or “Inspect Element”. Go to the “Console” tab. If your JavaScript is running without issues, there shouldn’t be any red error messages related to your script.
How do I ensure my JavaScript is mobile responsive?
Test your scripts on various devices and screen sizes. Also, consider using responsive frameworks like Bootstrap to ensure compatibility.
Is it safe to add third-party JavaScript to my WordPress site?
Always ensure that any third-party scripts come from reputable sources. Malicious scripts can compromise your website’s security.
Can I use JavaScript libraries like jQuery with WordPress?
Yes, WordPress comes with jQuery. However, ensure it’s enqueued correctly to avoid conflicts.
How do I handle JavaScript dependencies in WordPress?
WordPress’s wp_enqueue_script
function allows you to specify dependencies, ensuring scripts load in the correct order.
Why is my JavaScript slowing down my website?
Heavy scripts, especially those that aren’t optimized or minified, can slow down your website. Always optimize and prioritize loading essential scripts first.
Can I use asynchronous or deferred loading for my JavaScript?
Yes, this can help improve page load times by delaying the loading of non-essential scripts.
How do I troubleshoot JavaScript errors in WordPress?
The browser’s console is a great place to start. Additionally, consider using debugging tools and plugins to identify and resolve issues.
Your blog is a ray of sunshine in a sometimes dark and dreary world Thank you for spreading positivity and light
certainly like your website but you need to take a look at the spelling on quite a few of your posts Many of them are rife with spelling problems and I find it very troublesome to inform the reality nevertheless I will definitely come back again.
Usually I do not read article on blogs however I would like to say that this writeup very compelled me to take a look at and do it Your writing style has been amazed me Thank you very nice article
I was recommended this website by my cousin I am not sure whether this post is written by him as nobody else know such detailed about my trouble You are amazing Thanks.